A multi step form is a long form broken down in mutiple steps. It eliminates the problem of filling long form on landing page.
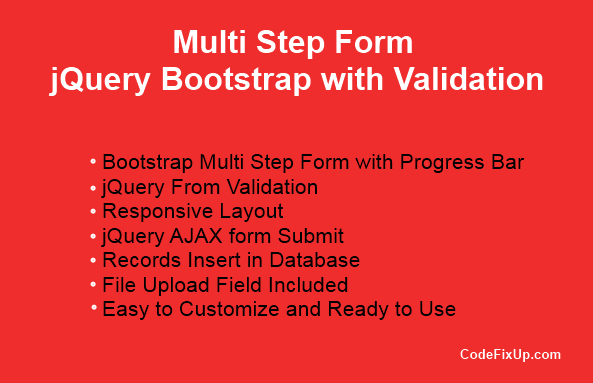
In this blog, we are going to explain, how to create responsive Multi Step Form jQuery Bootstrap with validation step by step process.
This tutorial provides a live demo of multi-step form wizard with field validation. This form also submits via jQuery ajax code and inserts data in the MySQL database. You can easily customize this form code and add bootstrap CSS ap per your requirement.
Steps to create Multi Step Form Widget:
- Create HTML form
- Add jQuery and Bootstrap
- Write Next and Previous Step jQuery Code
- Fields validation and Submit the form via Ajax
- Create MySQL Database and Insert Form data in it
Start creating jQuery Multi Step Form with Bootstrap
Lets check it out step by step process of create multi step form.
Step 1: Create HTML form
First index.html file and write down below HTML form code in it. This html form using bootstrap css element.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 |
<div class="container"> <div class="row"> <div class="col-xs-12" style="padding-right:0px;padding-left:0px;"> <div class="col-md-10 col-xs-12 col-md-offset-1"> <!-- multistep form --> <form class="form-horizontal form" id="msform" method="POST" action="" enctype="multipart/form-data"> <!-- progressbar --> <ul id="progressbar"> <li class="active">Step One</li> <li>Step Two</li> <li>Step Three</li> <li>Final Step</li> </ul> <div id="sucessmsg" style="display:none;"><h2 class="fs-subtitle" style="color: #dc3c52; font-size:22px; text-align:center;">Form Submitted Successfully</h2></div> <div id="errormsg" style="display:none;"><h2 class="fs-subtitle" style="color: #dc3c52; font-size:22px; text-align:center;">Oops.. Something wrong.</h2></div> <!-- fieldsets --> <fieldset id="step1"> <div align="center"> <h3 class="fs-subtitle">Multi Step form</h3> </div> <h2 class="fs-title">Personal Details</h2> <div class="form-group required"> <label class="control-label col-sm-2">First Name: </label> <div class="col-sm-10"> <input type="text" name="firstname"/> </div> </div> <div class="form-group required"> <label class="control-label col-sm-2">Last Names: </label> <div class="col-sm-10"> <input type="text" name="lastname" /> </div> </div> <div class="form-group required"> <label class="control-label col-sm-2">Gender:</label> <div class="col-sm-10"> <label class="checkstyle">Male <input type="radio" name="gender" value="Male"> <span class="checkmark"></span> </label> <label class="checkstyle">Female <input type="radio" name="gender" value="Female"> <span class="checkmark"></span> </label> <label class="checkstyle">Other <input type="radio" name="gender" value="Other"> <span class="checkmark"></span> </label> <label for="gender" class="error" generated="true"></label> </div> </div> <input style="float:right;" type="button" id="stepone" name="next" class="next action-button" value="Next" /> </fieldset> <fieldset id="step2"> <h2 class="fs-title">Contact Information</h2> <div class="form-group required"> <label class="control-label col-sm-2">Email: </label> <div class="col-sm-10"> <input type="text" name="email"/> </div> </div> <div class="form-group required"> <label class="control-label col-sm-2">Mobile Number: </label> <div class="col-sm-10"> <input type="text" name="mobilenumber"/> </div> </div> <input type="button" name="previous" id="previous1" class="previous action-button" value="Previous" /> <input style="float:right;" type="button" id="steptwo" name="next" class="next action-button" value="Next" /> </fieldset> <fieldset id="step3"> <h2 class="fs-title">Address Information</h2> <div class="form-group required"> <label class="control-label col-sm-2">Address: </label> <div class="col-sm-10"> <input type="text" name="address"/> </div> </div> <div class="form-group required"> <label class="control-label col-sm-2">Postal Code: </label> <div class="col-sm-10"> <input type="text" name="postalcode"/> </div> </div> <div class="form-group required"> <label class="control-label col-sm-2">City Name: </label> <div class="col-sm-10"> <input type="text" name="cityname"/> </div> </div> <input type="button" name="previous" id="previous2" class="previous action-button" value="Previous" /> <input style="float:right;" type="button" id="stepthree" name="next" class="next action-button" value="Next" /> </fieldset> <fieldset id="step4"> <h2 class="fs-title">Upload Document</h2> <div class="form-group"> <label class="control-label col-sm-2">Upload File: </label> <div class="col-sm-5"> <input type="file" name="uploadFile"> </div> </div> <div class="form-group"> <div class="col-sm-10"> <a href = "#" style="text-decoration: none;" class="terms_text"> <label style="padding-left: 30px;" class="checkstyle">I have read, agree and accept the terms and conditions <input type="checkbox" id="termscls" name="termsclsname"> <span class="checkmark"></span></a> </label> <label id="temsandconderror" style="color:red;display:none;">Please Accept Terms & Conditions</label> </div> </div> <input type="button" name="previous" id="previous3" class="previous action-button" value="Previous" /> <input style="float:right;" type="submit" id="stepfour" name="submit" class="submitbutton" value="Submit" /> </fieldset> </form> </div> </div> </div> </div> |
Step 2: Add jQuery, Bootstrap, and Style CSS files
Now add validate jQuery and Boostrap CSS and JS file in index.html file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<!-- Google Font --> <link href='https://fonts.googleapis.com/css?family=Source+Sans+Pro:400,300,600,200' rel='stylesheet' type='text/css'> <!-- Stylesheets --> <link rel="stylesheet" href="css/style.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <script src="https://code.jquery.com/jquery-3.4.1.min.js" type="text/javascript"></script> <script src="js/jquery.validate.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-easing/1.3/jquery.easing.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script> |
Add Style.css code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 |
html { height: 100%; /* background-color: #e3e9ee; */ } html, body { font-family: 'Source Sans Pro', sans-serif; font-size: 14px; width: 100%; } a { color: #d91b5b; text-decoration: none; font-weight: 600; padding-bottom: 3px; -webkit-transition: all 0.2s; -moz-transition: all 0.2s; transition: all 0.2s; } a:hover { border-bottom: 1px solid; } .navbar-brand > img{ max-width:240px; } /* Form styles */ #msform { width: 100%; margin: 50px auto; position: relative; } #msform fieldset { background: #fff; border: 0 none; border-radius: 5px; box-sizing: border-box; width: 100%; margin: 0 0% 20px; /*stacking fieldsets above each other*/ position: relative; } /* Hide all except first fieldset */ #msform fieldset:not(:first-of-type) { display: none; } img.logo { max-width: 155px; margin-top: 5px; } #msform p { color: #8b9ab0; font-size: 12px; } #msform label { padding-right:0px 15px; font-size: 16px; text-align: left; /* font-weight:600px !important; */ } /* Inputs */ #msform input, #msform textarea { padding: 5px 15px; border: 1px solid transparent; border-radius: 3px; margin-bottom: 10px; margin-top: 5px; background-color: #eef5ff; width: 100%; box-sizing: border-box; font-family: montserrat; color: #333; font-size: 14px; font-family: inherit; } #msform input:focus, #msform textarea:focus { outline: none; border-color: #7bbdf3; } /* Buttons */ #msform .submitbutton { width: 30%; text-transform: uppercase; background: #d91b5b; font-weight: bold; color: white; border: 1px solid transparent; border-radius: 3px; cursor: pointer; padding: 12px 5px; margin: 10px 0; font-size: 16px; display: inline-block; -webkit-transition: all 0.2s; -moz-transition: all 0.2s; transition: all 0.2s; } #msform .action-button { width: 30%; text-transform: uppercase; background: #d91b5b; font-weight: bold; color: white; border: 1px solid transparent; border-radius: 3px; cursor: pointer; padding: 12px 5px; margin: 10px 0; font-size: 16px; display: inline-block; -webkit-transition: all 0.2s; -moz-transition: all 0.2s; transition: all 0.2s; } #msform .previous.action-button { background: #fff; border: 1px solid #7bbdf3; color: #7bbdf3; } #msform .action-button:hover, #msform .action-button:focus { box-shadow: 0 10px 30px 1px rgba(0, 0, 0, 0.2); } /* Headings */ .fs-title { font-size: 20px; font-weight: 400; color: #a94442; margin-bottom: 20px; background-color: #9999CC; margin-top: 20px; padding:5px; color:#fff; } .fs-subtitle { font-weight: 400; font-size: 19px; color: #434a54; margin-bottom: 20px; } /* Progressbar */ #progressbar { margin-bottom: 30px; overflow: hidden; /*CSS counters to number the steps*/ counter-reset: step; } #progressbar li { list-style-type: none; color: #8b9ab0; text-transform: uppercase; font-size: 9px; width: 25%; float: left; position: relative; text-align: center; } #progressbar li.active { color: #d91b5b; } #progressbar li:before { content: counter(step); counter-increment: step; width: 20px; line-height: 20px; display: block; font-size: 10px; color: #333; background: white; border-radius: 3em; margin: 0 auto 5px auto; text-align: center; } /* Progressbar connectors */ #progressbar li:after { content: ''; width: 100%; height: 2px; background: white; position: absolute; left: -50%; top: 9px; z-index: -1; } #progressbar li:first-child:after { /* connector not needed before the first step */ content: none; } /* Marking active/completed steps green */ /*The number of the step and the connector before it = green*/ #progressbar li.active:before, #progressbar li.active:after{ background: #d91b5b; color: white; } /* css for checkbox */ /* The container */ #msform .checkstyle { display: inline-flex; position: relative; width: auto; padding-left: 35px; padding-right: 25px; margin-bottom: 12px; cursor: pointer; font-size: 16px; -webkit-user-select: none; -moz-user-select: none; -ms-user-select: none; user-select: none; } /* Hide the browser's default checkbox */ #msform .checkstyle input { position: absolute; opacity: 0; cursor: pointer; height: 0; width: 0; } /* Create a custom checkbox */ #msform .checkmark { position: absolute; top: 0; left: 0; height: 25px; width: 25px; background-color: #eee; } /* On mouse-over, add a grey background color */ #msform .checkstyle:hover input ~ .checkmark { background-color: #ccc; } /* When the checkbox is checked, add a blue background */ #msform .checkstyle input:checked ~ .checkmark { background-color: #2196F3; } /* Create the checkmark/indicator (hidden when not checked) */ #msform .checkmark:after { content: ""; position: absolute; display: none; } /* Show the checkmark when checked */ #msform .checkstyle input:checked ~ .checkmark:after { display: block; } /* Style the checkmark/indicator */ #msform .checkstyle .checkmark:after { left: 9px; top: 5px; width: 5px; height: 10px; border: solid white; border-width: 0 3px 3px 0; -webkit-transform: rotate(45deg); -ms-transform: rotate(45deg); transform: rotate(45deg); } .terms_text a:hover { text-decoration:none !important; } .listordercls { line-height: 25px !important; font-size:13px !important; list-style: none !important; padding-left:0px !important; } /* Header */ header { margin: 0; padding: 0; position: sticky; position: -webkit-sticky; top: 0; z-index: 999; border-bottom: 1px solid #00000024; } header .navbar { margin: 0; padding: 0; background-color: #fff; border-radius: 0px; } /* Footer */ footer { margin: 0; padding: 55px 0 50px; float: left; width: 100%; text-align: center; } footer img { max-height: 60px; margin-bottom: 35px; } .footer-img2 { margin-left: auto; margin-right: auto; display: table; width: auto; float: none; } .footer-img3 { float: right; } footer p { margin-bottom: 2px; color: #272727; font-size: 13px; } footer a { color: #272727; font-size: 13px; } @media (max-width:480px){ .navbar-brand > img{ max-width:180px !important; } #enroltextcls{ font-size: 14px !important; } } |
Step 3: Write Next and Previous Step jQuery Code
Write down jQuery code to add Next and Previous step moving functionality. ( v.form() belongs to your current HTML form id.)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
$("#stepone").click(function() { current_fs = $(this).parent(); next_fs = $(this).parent().next(); if (v.form()) { $("input","#step2").removeClass("ignore"); $("#progressbar li").eq($("fieldset").index(next_fs)).addClass("active"); $('#step1').hide(); $('#step2').show(); window.scrollTo(0, 0); } }); $("#previous1").click(function() { $("input","#step2").addClass("ignore"); current_fs = $(this).parent(); previous_fs = $(this).parent().prev(); $("#progressbar li").eq($("fieldset").index(current_fs)).removeClass("active"); $('#step2').hide(); $('#step1').show(); window.scrollTo(0, 0); }); |
Step 4: Fields validation and Submit form via Ajax
Now add form fields jQuery validation code and ajax form submit functionality.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
// validate form on keyup and submit var v = jQuery("#msform").validate({ ignore: ".ignore", rules: { firstname: "required", lastname: "required", 'gender': { required: true }, address: "required", mobilenumber: "required", email: "required", postalcode: "required", cityname: "required" }, submitHandler: function(form) { var formData = new FormData(form); //e.preventDefault(); $("#loadingmessage").show(); $.ajax({ url: "formsubmitajax.php", type: "POST", data: formData, contentType: false, cache: false, processData:false, success: function(data) { if(data == 'success') { $("#loadingmessage").hide(); $("#sucessmsg").show(); } if(data == 'error') { $("#loadingmessage").hide(); $("#errormsg").show(); } }, error: function(){} }); //return false; //This doesn't prevent the form from submitting. }, highlight: function(element, errorClass) { window.scrollTo(0, 0); }, unhighlight: function(element, errorClass) { //$(element).closest(".form-group").removeClass("has-error"); }, }); |
Step 5: Create MySQL Database and Insert Form data in it
First, create a MySql database table to insert form submit data. Use the below MySql command to create the new table.
1 2 3 4 5 6 7 8 9 10 11 12 |
CREATE TABLE `multi_step_form_data` ( `id` int(11) NOT NULL, `firstname` varchar(256) NOT NULL, `lastname` varchar(256) NOT NULL, `gender` varchar(256) NOT NULL, `email` varchar(256) NOT NULL, `mobilenumber` varchar(256) NOT NULL, `address` varchar(256) NOT NULL, `postalcode` varchar(256) NOT NULL, `cityname` varchar(256) NOT NULL, `filename` varchar(256) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; |
Now create formsubmitajax.php file to handle form submit AJAX call.
Note: Also create database.php file to make the MySqli database connection and include in formsubmitajax.php file, so insert sql query will work in it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
<?php require_once "database.php"; // save data in database // prepare and bind $stmt = $link->prepare("INSERT INTO multi_step_form_data (firstname, lastname, gender, email, mobilenumber, address, postalcode, cityname, filename) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("sssssssss", $firstname, $lastname, $gender, $email, $mobilenumber, $address, $postalcode, $cityname, $DOBFileDocname); /* Set the parameters values and execute the statement again to insert another row */ $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $gender = $_POST['gender']; $email = $_POST['email']; $mobilenumber = $_POST['mobilenumber']; $address = $_POST['address']; $postalcode = $_POST['postalcode']; $cityname = $_POST['cityname']; $thirdntarget_dir = "uploadedFiles/"; $DOBFileDocname = $_FILES["uploadFile"]["name"]; $thirdntarget_dir = $thirdntarget_dir.$DOBFileDocname; move_uploaded_file($_FILES["uploadFile"]["tmp_name"], $thirdntarget_dir); //$stmt->execute(); //echo "New records created successfully"; if($stmt->execute()) { echo "success"; } else { echo "error"; } $stmt->close(); $link->close(); ?> |
That’s it. You can check the live demo to see how the jQuery multi step form works. This is a completely responsive form and ready to use.